CampNight
Year: 2022
Genre: Survival
Platform: Windows PC
GitHub
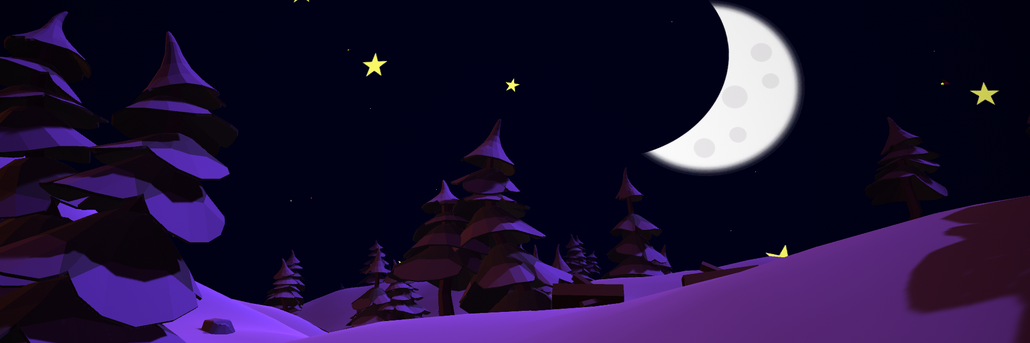
CampNight is my first indie game that I completed from start to finish and released on Steam. The game has been very well received and is rated 'positive' on the platform. On this page I want to show some of the features.
Terrain generation
When I started working on the project, I didn't have any plans for it. I just wanted to make a survival game with random terrain generation. Later I choose for the snow enviroment.
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.AI;
public class TerrainGeneration : MonoBehaviour
{
Mesh mesh;
Vector3 offsetPosition;
Vector3[] vertices;
public int xSize;
public int zSize;
public int seed;
[Range(1,10)]public int octaves;
public float frequencyMultiplier;
public float amplitudeMultiplier;
public float heightMultiplier;
public float center;
public float fallOff;
float fallOffX;
float fallOffZ;
float fallOffXZ;
int[] triangles;
public GameObject gameEvents;
public NavMeshSurface[] surfaces;
// Start is called before the first frame update
public void Start()
{
if (seed == 0)
{
seed = Random.Range(0, 99999);
}
mesh = new Mesh();
GetComponent<MeshFilter>().mesh = mesh;
CreateShape();
UpdateMesh();
}
//generates a random terrain using a seed
void CreateShape()
{
vertices = new Vector3[(xSize + 1) * (zSize + 1)];
int i = 0;
for (int z = 0; z <= zSize; z++)
{
for (int x = 0; x <= xSize; x++)
{
float freq = 1;
float amp = 1;
float noieHeight = 0;
for (int j = 0; j < octaves; j++)
{
fallOffX = (center * center - 2 * center * x + 4 * heightMultiplier
* fallOff + x * x) / 4 * fallOff;
fallOffZ = (center * center - 2 * center * z + 4 * heightMultiplier
* fallOff + z * z) / 4 * fallOff;
fallOffXZ = (fallOffX + fallOffZ) / 2;
noieHeight += (Mathf.PerlinNoise((x * .03f * freq) + seed,
(z * .03f * freq) + seed) * heightMultiplier * amp) - fallOffXZ;
amp *= amplitudeMultiplier;
freq *= frequencyMultiplier;
}
float y = noieHeight;
if (y < 8.4f)
{
y = 8.4f;
}
vertices[i] = new Vector3(x, y, z);
i++;
}
}
triangles = new int[xSize * zSize * 6];
int vert = 0;
int tris = 0;
for (int z = 0; z < zSize; z++)
{
for (int x = 0; x < xSize; x++)
{
triangles[tris + 0] = vert + 0;
triangles[tris + 1] = vert + xSize + 1;
triangles[tris + 2] = vert + 1;
triangles[tris + 3] = vert + 1;
triangles[tris + 4] = vert + xSize + 1;
triangles[tris + 5] = vert + xSize + 2;
vert++;
tris += 6;
}
vert++;
}
}
void UpdateMesh()
{
mesh.Clear();
mesh.vertices = vertices;
mesh.triangles = triangles;
mesh.RecalculateNormals();
GetComponent<MeshCollider>().sharedMesh = mesh;
for (int i = 0; i < surfaces.Length; i++)
{
surfaces[i].BuildNavMesh();
}
}
}